pathlib
— Object-oriented filesystem paths¶
Added in version 3.4.
Source code: Lib/pathlib.py
Цей модуль пропонує класи, що представляють шляхи файлової системи із семантикою, відповідною до різних операційних систем. Класи шляхів поділяються на чисті шляхи, які забезпечують суто обчислювальні операції без введення/виведення, та конкретні шляхи, які успадковують чисті шляхи, але також забезпечують операції введення/виведення.
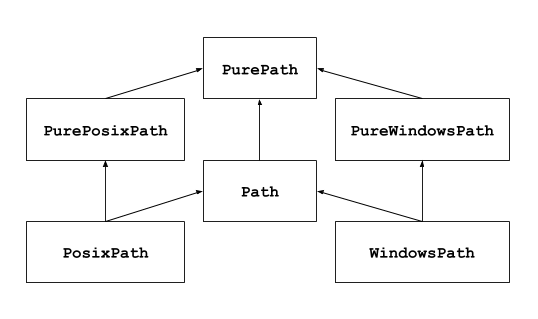
Якщо ви ніколи раніше не користувалися цим модулем або просто не впевнені, який клас підходить для вашого завдання, Path
, швидше за все, це те, що вам потрібно. Він створює екземпляр конкретного шляху для платформи, на якій працює код.
Чисті шляхи корисні в деяких особливих випадках; наприклад:
Якщо ви хочете маніпулювати шляхами Windows на машині Unix (або навпаки). Ви не можете створити екземпляр
WindowsPath
під час роботи в Unix, але ви можете створити екземплярPureWindowsPath
.Ви хочете переконатися, що ваш код обробляє лише шляхи без фактичного доступу до ОС. У цьому випадку створення одного з чистих класів може бути корисним, оскільки вони просто не мають жодних операцій доступу до ОС.
Дивись також
PEP 428: Модуль pathlib – шляхи об’єктно-орієнтованої файлової системи.
Дивись також
Для низькорівневих маніпуляцій шляхом до рядків ви також можете використовувати модуль os.path
.
Основне використання¶
Імпорт основного класу:
>>> from pathlib import Path
Перелік підкаталогів:
>>> p = Path('.')
>>> [x for x in p.iterdir() if x.is_dir()]
[PosixPath('.hg'), PosixPath('docs'), PosixPath('dist'),
PosixPath('__pycache__'), PosixPath('build')]
Перелік вихідних файлів Python у цьому дереві каталогів:
>>> list(p.glob('**/*.py'))
[PosixPath('test_pathlib.py'), PosixPath('setup.py'),
PosixPath('pathlib.py'), PosixPath('docs/conf.py'),
PosixPath('build/lib/pathlib.py')]
Навігація всередині дерева каталогів:
>>> p = Path('/etc')
>>> q = p / 'init.d' / 'reboot'
>>> q
PosixPath('/etc/init.d/reboot')
>>> q.resolve()
PosixPath('/etc/rc.d/init.d/halt')
Властивості шляху запиту:
>>> q.exists()
True
>>> q.is_dir()
False
Відкриття файлу:
>>> with q.open() as f: f.readline()
...
'#!/bin/bash\n'
Чисті стежки¶
Об’єкти чистого шляху забезпечують операції обробки шляху, які фактично не мають доступу до файлової системи. Є три способи отримати доступ до цих класів, які ми також називаємо смаками:
- class pathlib.PurePath(*pathsegments)¶
Загальний клас, який представляє шлях до системи (його екземпляр створює або
PurePosixPath
, абоPureWindowsPath
):>>> PurePath('setup.py') # Running on a Unix machine PurePosixPath('setup.py')
Each element of pathsegments can be either a string representing a path segment, or an object implementing the
os.PathLike
interface where the__fspath__()
method returns a string, such as another path object:>>> PurePath('foo', 'some/path', 'bar') PurePosixPath('foo/some/path/bar') >>> PurePath(Path('foo'), Path('bar')) PurePosixPath('foo/bar')
Якщо pathsegments порожній, передбачається поточний каталог:
>>> PurePath() PurePosixPath('.')
If a segment is an absolute path, all previous segments are ignored (like
os.path.join()
):>>> PurePath('/etc', '/usr', 'lib64') PurePosixPath('/usr/lib64') >>> PureWindowsPath('c:/Windows', 'd:bar') PureWindowsPath('d:bar')
On Windows, the drive is not reset when a rooted relative path segment (e.g.,
r'\foo'
) is encountered:>>> PureWindowsPath('c:/Windows', '/Program Files') PureWindowsPath('c:/Program Files')
Фальшиві косі риски та одиночні крапки згортаються, але подвійні крапки (
'..''
) і початкові подвійні косі риски ('//'
) – ні, оскільки це може змінити значення шляху з різних причин (наприклад, символічні посилання, шляхи UNC):>>> PurePath('foo//bar') PurePosixPath('foo/bar') >>> PurePath('//foo/bar') PurePosixPath('//foo/bar') >>> PurePath('foo/./bar') PurePosixPath('foo/bar') >>> PurePath('foo/../bar') PurePosixPath('foo/../bar')
(наївний підхід зробив би
PurePosixPath('foo/../bar')
еквівалентнимPurePosixPath('bar')
, що є неправильним, якщоfoo
є символічним посиланням на інший каталог )Об’єкти чистого шляху реалізують інтерфейс
os.PathLike
, що дозволяє використовувати їх будь-де, де прийнятний інтерфейс.Змінено в версії 3.6: Додано підтримку інтерфейсу
os.PathLike
.
- class pathlib.PurePosixPath(*pathsegments)¶
Підклас
PurePath
, цей варіант шляху представляє шляхи до файлової системи не Windows:>>> PurePosixPath('/etc/hosts') PurePosixPath('/etc/hosts')
pathsegments вказується подібно до
PurePath
.
- class pathlib.PureWindowsPath(*pathsegments)¶
Підклас
PurePath
, цей варіант шляху представляє шляхи файлової системи Windows, включаючи шляхи UNC:>>> PureWindowsPath('c:/', 'Users', 'Ximénez') PureWindowsPath('c:/Users/Ximénez') >>> PureWindowsPath('//server/share/file') PureWindowsPath('//server/share/file')
pathsegments вказується подібно до
PurePath
.
Незалежно від системи, на якій ви працюєте, ви можете створити екземпляри всіх цих класів, оскільки вони не забезпечують жодних операцій, які виконують системні виклики.
Загальні властивості¶
Paths are immutable and hashable. Paths of a same flavour are comparable and orderable. These properties respect the flavour’s case-folding semantics:
>>> PurePosixPath('foo') == PurePosixPath('FOO')
False
>>> PureWindowsPath('foo') == PureWindowsPath('FOO')
True
>>> PureWindowsPath('FOO') in { PureWindowsPath('foo') }
True
>>> PureWindowsPath('C:') < PureWindowsPath('d:')
True
Шляхи різного смаку порівнюються нерівнозначно і не можуть бути впорядковані:
>>> PureWindowsPath('foo') == PurePosixPath('foo')
False
>>> PureWindowsPath('foo') < PurePosixPath('foo')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: '<' not supported between instances of 'PureWindowsPath' and 'PurePosixPath'
Оператори¶
The slash operator helps create child paths, like os.path.join()
.
If the argument is an absolute path, the previous path is ignored.
On Windows, the drive is not reset when the argument is a rooted
relative path (e.g., r'\foo'
):
>>> p = PurePath('/etc')
>>> p
PurePosixPath('/etc')
>>> p / 'init.d' / 'apache2'
PurePosixPath('/etc/init.d/apache2')
>>> q = PurePath('bin')
>>> '/usr' / q
PurePosixPath('/usr/bin')
>>> p / '/an_absolute_path'
PurePosixPath('/an_absolute_path')
>>> PureWindowsPath('c:/Windows', '/Program Files')
PureWindowsPath('c:/Program Files')
Об’єкт шляху можна використовувати скрізь, де прийнятний об’єкт, що реалізує os.PathLike
:
>>> import os
>>> p = PurePath('/etc')
>>> os.fspath(p)
'/etc'
Рядкове представлення шляху — це сам необроблений шлях до файлової системи (у рідній формі, наприклад із зворотними похилими рисками під Windows), який ви можете передати будь-якій функції, яка приймає шлях до файлу як рядок:
>>> p = PurePath('/etc')
>>> str(p)
'/etc'
>>> p = PureWindowsPath('c:/Program Files')
>>> str(p)
'c:\\Program Files'
Подібним чином виклик bytes
для шляху дає необроблений шлях до файлової системи як об’єкт bytes, закодований os.fsencode()
:
>>> bytes(p)
b'/etc'
Примітка
Виклик bytes
рекомендовано лише в Unix. У Windows форма Юнікод є канонічним представленням шляхів файлової системи.
Доступ до окремих частин¶
Щоб отримати доступ до окремих «частин» (компонентів) шляху, використовуйте наступну властивість:
- PurePath.parts¶
Кортеж, що надає доступ до різних компонентів шляху:
>>> p = PurePath('/usr/bin/python3') >>> p.parts ('/', 'usr', 'bin', 'python3') >>> p = PureWindowsPath('c:/Program Files/PSF') >>> p.parts ('c:\\', 'Program Files', 'PSF')
(зверніть увагу, як диск і локальний корінь перегруповані в одній частині)
Методи та властивості¶
Чисті шляхи забезпечують такі методи та властивості:
- PurePath.drive¶
Рядок, що представляє літеру або назву диска, якщо є:
>>> PureWindowsPath('c:/Program Files/').drive 'c:' >>> PureWindowsPath('/Program Files/').drive '' >>> PurePosixPath('/etc').drive ''
Акції UNC також вважаються накопичувачами:
>>> PureWindowsPath('//host/share/foo.txt').drive '\\\\host\\share'
- PurePath.root¶
Рядок, що представляє (локальний або глобальний) корінь, якщо такий є:
>>> PureWindowsPath('c:/Program Files/').root '\\' >>> PureWindowsPath('c:Program Files/').root '' >>> PurePosixPath('/etc').root '/'
Акції UNC завжди мають корінь:
>>> PureWindowsPath('//host/share').root '\\'
Якщо шлях починається з більш ніж двох послідовних похилих рисок,
PurePosixPath
їх згортає:>>> PurePosixPath('//etc').root '//' >>> PurePosixPath('///etc').root '/' >>> PurePosixPath('////etc').root '/'
Примітка
This behavior conforms to The Open Group Base Specifications Issue 6, paragraph 4.11 Pathname Resolution:
«Ім’я шляху, що починається з двох послідовних похилих рисок, може інтерпретуватися у спосіб, визначений реалізацією, хоча більше двох початкових похилих рисок повинні розглядатися як одна похила риска.»
- PurePath.anchor¶
Конкатенація диска та кореня:
>>> PureWindowsPath('c:/Program Files/').anchor 'c:\\' >>> PureWindowsPath('c:Program Files/').anchor 'c:' >>> PurePosixPath('/etc').anchor '/' >>> PureWindowsPath('//host/share').anchor '\\\\host\\share\\'
- PurePath.parents¶
Незмінна послідовність, що забезпечує доступ до логічних предків шляху:
>>> p = PureWindowsPath('c:/foo/bar/setup.py') >>> p.parents[0] PureWindowsPath('c:/foo/bar') >>> p.parents[1] PureWindowsPath('c:/foo') >>> p.parents[2] PureWindowsPath('c:/')
Змінено в версії 3.10: Батьківська послідовність тепер підтримує slices і від’ємні значення індексу.
- PurePath.parent¶
Логічний батько шляху:
>>> p = PurePosixPath('/a/b/c/d') >>> p.parent PurePosixPath('/a/b/c')
Ви не можете пройти повз якір або порожній шлях
>>> p = PurePosixPath('/') >>> p.parent PurePosixPath('/') >>> p = PurePosixPath('.') >>> p.parent PurePosixPath('.')
Примітка
Це суто лексична операція, тому така поведінка:
>>> p = PurePosixPath('foo/..') >>> p.parent PurePosixPath('foo')
If you want to walk an arbitrary filesystem path upwards, it is recommended to first call
Path.resolve()
so as to resolve symlinks and eliminate".."
components.
- PurePath.name¶
Рядок, що представляє кінцевий компонент шляху, за винятком диска та кореня, якщо такі є:
>>> PurePosixPath('my/library/setup.py').name 'setup.py'
Назви дисків UNC не враховуються:
>>> PureWindowsPath('//some/share/setup.py').name 'setup.py' >>> PureWindowsPath('//some/share').name ''
- PurePath.suffix¶
The file extension of the final component, if any:
>>> PurePosixPath('my/library/setup.py').suffix '.py' >>> PurePosixPath('my/library.tar.gz').suffix '.gz' >>> PurePosixPath('my/library').suffix ''
- PurePath.suffixes¶
A list of the path’s file extensions:
>>> PurePosixPath('my/library.tar.gar').suffixes ['.tar', '.gar'] >>> PurePosixPath('my/library.tar.gz').suffixes ['.tar', '.gz'] >>> PurePosixPath('my/library').suffixes []
- PurePath.stem¶
Остаточний компонент шляху без суфікса:
>>> PurePosixPath('my/library.tar.gz').stem 'library.tar' >>> PurePosixPath('my/library.tar').stem 'library' >>> PurePosixPath('my/library').stem 'library'
- PurePath.as_posix()¶
Повертає рядкове представлення шляху з похилою рискою (
/
):>>> p = PureWindowsPath('c:\\windows') >>> str(p) 'c:\\windows' >>> p.as_posix() 'c:/windows'
- PurePath.as_uri()¶
Represent the path as a
file
URI.ValueError
is raised if the path isn’t absolute.>>> p = PurePosixPath('/etc/passwd') >>> p.as_uri() 'file:///etc/passwd' >>> p = PureWindowsPath('c:/Windows') >>> p.as_uri() 'file:///c:/Windows'
- PurePath.is_absolute()¶
Повернути, чи є шлях абсолютним чи ні. Шлях вважається абсолютним, якщо він має як корінь, так і (якщо дозволяє аромат) диск:
>>> PurePosixPath('/a/b').is_absolute() True >>> PurePosixPath('a/b').is_absolute() False >>> PureWindowsPath('c:/a/b').is_absolute() True >>> PureWindowsPath('/a/b').is_absolute() False >>> PureWindowsPath('c:').is_absolute() False >>> PureWindowsPath('//some/share').is_absolute() True
- PurePath.is_relative_to(other)¶
Повертає, чи є цей шлях відносно іншого шляху.
>>> p = PurePath('/etc/passwd') >>> p.is_relative_to('/etc') True >>> p.is_relative_to('/usr') False
This method is string-based; it neither accesses the filesystem nor treats «
..
» segments specially. The following code is equivalent:>>> u = PurePath('/usr') >>> u == p or u in p.parents False
Added in version 3.9.
Deprecated since version 3.12, will be removed in version 3.14: Passing additional arguments is deprecated; if supplied, they are joined with other.
- PurePath.is_reserved()¶
За допомогою
PureWindowsPath
повернітьTrue
, якщо шлях вважається зарезервованим під Windows,False
інакше. ЗPurePosixPath
завжди повертаєтьсяFalse
.>>> PureWindowsPath('nul').is_reserved() True >>> PurePosixPath('nul').is_reserved() False
File system calls on reserved paths can fail mysteriously or have unintended effects.
- PurePath.joinpath(*pathsegments)¶
Calling this method is equivalent to combining the path with each of the given pathsegments in turn:
>>> PurePosixPath('/etc').joinpath('passwd') PurePosixPath('/etc/passwd') >>> PurePosixPath('/etc').joinpath(PurePosixPath('passwd')) PurePosixPath('/etc/passwd') >>> PurePosixPath('/etc').joinpath('init.d', 'apache2') PurePosixPath('/etc/init.d/apache2') >>> PureWindowsPath('c:').joinpath('/Program Files') PureWindowsPath('c:/Program Files')
- PurePath.match(pattern, *, case_sensitive=None)¶
Match this path against the provided glob-style pattern. Return
True
if matching is successful,False
otherwise.If pattern is relative, the path can be either relative or absolute, and matching is done from the right:
>>> PurePath('a/b.py').match('*.py') True >>> PurePath('/a/b/c.py').match('b/*.py') True >>> PurePath('/a/b/c.py').match('a/*.py') False
If pattern is absolute, the path must be absolute, and the whole path must match:
>>> PurePath('/a.py').match('/*.py') True >>> PurePath('a/b.py').match('/*.py') False
The pattern may be another path object; this speeds up matching the same pattern against multiple files:
>>> pattern = PurePath('*.py') >>> PurePath('a/b.py').match(pattern) True
Примітка
The recursive wildcard «
**
» isn’t supported by this method (it acts like non-recursive «*
».)Змінено в версії 3.12: Accepts an object implementing the
os.PathLike
interface.Як і в інших методах, чутливість до регістру відповідає стандартам платформи:
>>> PurePosixPath('b.py').match('*.PY') False >>> PureWindowsPath('b.py').match('*.PY') True
Set case_sensitive to
True
orFalse
to override this behaviour.Змінено в версії 3.12: The case_sensitive parameter was added.
- PurePath.relative_to(other, walk_up=False)¶
Compute a version of this path relative to the path represented by other. If it’s impossible,
ValueError
is raised:>>> p = PurePosixPath('/etc/passwd') >>> p.relative_to('/') PurePosixPath('etc/passwd') >>> p.relative_to('/etc') PurePosixPath('passwd') >>> p.relative_to('/usr') Traceback (most recent call last): File "<stdin>", line 1, in <module> File "pathlib.py", line 941, in relative_to raise ValueError(error_message.format(str(self), str(formatted))) ValueError: '/etc/passwd' is not in the subpath of '/usr' OR one path is relative and the other is absolute.
When walk_up is false (the default), the path must start with other. When the argument is true,
..
entries may be added to form the relative path. In all other cases, such as the paths referencing different drives,ValueError
is raised.:>>> p.relative_to('/usr', walk_up=True) PurePosixPath('../etc/passwd') >>> p.relative_to('foo', walk_up=True) Traceback (most recent call last): File "<stdin>", line 1, in <module> File "pathlib.py", line 941, in relative_to raise ValueError(error_message.format(str(self), str(formatted))) ValueError: '/etc/passwd' is not on the same drive as 'foo' OR one path is relative and the other is absolute.
Попередження
This function is part of
PurePath
and works with strings. It does not check or access the underlying file structure. This can impact the walk_up option as it assumes that no symlinks are present in the path; callresolve()
first if necessary to resolve symlinks.Змінено в версії 3.12: The walk_up parameter was added (old behavior is the same as
walk_up=False
).Deprecated since version 3.12, will be removed in version 3.14: Passing additional positional arguments is deprecated; if supplied, they are joined with other.
- PurePath.with_name(name)¶
Повертає новий шлях зі зміненим
name
. Якщо вихідний шлях не має імені, виникає ValueError:>>> p = PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>> p.with_name('setup.py') PureWindowsPath('c:/Downloads/setup.py') >>> p = PureWindowsPath('c:/') >>> p.with_name('setup.py') Traceback (most recent call last): File "<stdin>", line 1, in <module> File "/home/antoine/cpython/default/Lib/pathlib.py", line 751, in with_name raise ValueError("%r has an empty name" % (self,)) ValueError: PureWindowsPath('c:/') has an empty name
- PurePath.with_stem(stem)¶
Повертає новий шлях зі зміненим
stem
. Якщо вихідний шлях не має імені, виникає ValueError:>>> p = PureWindowsPath('c:/Downloads/draft.txt') >>> p.with_stem('final') PureWindowsPath('c:/Downloads/final.txt') >>> p = PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>> p.with_stem('lib') PureWindowsPath('c:/Downloads/lib.gz') >>> p = PureWindowsPath('c:/') >>> p.with_stem('') Traceback (most recent call last): File "<stdin>", line 1, in <module> File "/home/antoine/cpython/default/Lib/pathlib.py", line 861, in with_stem return self.with_name(stem + self.suffix) File "/home/antoine/cpython/default/Lib/pathlib.py", line 851, in with_name raise ValueError("%r has an empty name" % (self,)) ValueError: PureWindowsPath('c:/') has an empty name
Added in version 3.9.
- PurePath.with_suffix(suffix)¶
Повертає новий шлях зі зміненим
suffix
. Якщо вихідний шлях не має суфікса, замість нього додається новий суфікс. Якщо суфікс є порожнім рядком, вихідний суфікс видаляється:>>> p = PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>> p.with_suffix('.bz2') PureWindowsPath('c:/Downloads/pathlib.tar.bz2') >>> p = PureWindowsPath('README') >>> p.with_suffix('.txt') PureWindowsPath('README.txt') >>> p = PureWindowsPath('README.txt') >>> p.with_suffix('') PureWindowsPath('README')
- PurePath.with_segments(*pathsegments)¶
Create a new path object of the same type by combining the given pathsegments. This method is called whenever a derivative path is created, such as from
parent
andrelative_to()
. Subclasses may override this method to pass information to derivative paths, for example:from pathlib import PurePosixPath class MyPath(PurePosixPath): def __init__(self, *pathsegments, session_id): super().__init__(*pathsegments) self.session_id = session_id def with_segments(self, *pathsegments): return type(self)(*pathsegments, session_id=self.session_id) etc = MyPath('/etc', session_id=42) hosts = etc / 'hosts' print(hosts.session_id) # 42
Added in version 3.12.
Бетонні доріжки¶
Конкретні шляхи є підкласами класів чистих шляхів. На додаток до операцій, наданих останнім, вони також надають методи для виконання системних викликів об’єктів шляху. Є три способи створення конкретних шляхів:
- class pathlib.Path(*pathsegments)¶
Підклас
PurePath
, цей клас представляє конкретні шляхи шляхів системи (інстанціювання створює абоPosixPath
, абоWindowsPath
):>>> Path('setup.py') PosixPath('setup.py')
pathsegments вказується подібно до
PurePath
.
- class pathlib.PosixPath(*pathsegments)¶
Підклас
Path
іPurePosixPath
, цей клас представляє конкретні шляхи до файлової системи не Windows:>>> PosixPath('/etc/hosts') PosixPath('/etc/hosts')
pathsegments вказується подібно до
PurePath
.
- class pathlib.WindowsPath(*pathsegments)¶
Підклас
Path
іPureWindowsPath
, цей клас представляє конкретні шляхи файлової системи Windows:>>> WindowsPath('c:/', 'Users', 'Ximénez') WindowsPath('c:/Users/Ximénez')
pathsegments вказується подібно до
PurePath
.
Ви можете створити лише екземпляр класу, який відповідає вашій системі (дозвол системних викликів за несумісними варіантами шляху може призвести до помилок або збоїв у вашій програмі):
>>> import os
>>> os.name
'posix'
>>> Path('setup.py')
PosixPath('setup.py')
>>> PosixPath('setup.py')
PosixPath('setup.py')
>>> WindowsPath('setup.py')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "pathlib.py", line 798, in __new__
% (cls.__name__,))
NotImplementedError: cannot instantiate 'WindowsPath' on your system
Some concrete path methods can raise an OSError
if a system call fails
(for example because the path doesn’t exist).
Expanding and resolving paths¶
- classmethod Path.home()¶
Повертає новий об’єкт шляху, що представляє домашній каталог користувача (як повертає
os.path.expanduser()
за допомогою конструкції~
). Якщо не вдається вирішити домашній каталог, виникаєRuntimeError
.>>> Path.home() PosixPath('/home/antoine')
Added in version 3.5.
- Path.expanduser()¶
Повертає новий шлях із розширеними конструкціями
~
і~user
, як повертаєos.path.expanduser()
. Якщо не вдається вирішити домашній каталог, виникаєRuntimeError
.>>> p = PosixPath('~/films/Monty Python') >>> p.expanduser() PosixPath('/home/eric/films/Monty Python')
Added in version 3.5.
- classmethod Path.cwd()¶
Повертає новий об’єкт шляху, що представляє поточний каталог (як повертає
os.getcwd()
):>>> Path.cwd() PosixPath('/home/antoine/pathlib')
- Path.absolute()¶
Make the path absolute, without normalization or resolving symlinks. Returns a new path object:
>>> p = Path('tests') >>> p PosixPath('tests') >>> p.absolute() PosixPath('/home/antoine/pathlib/tests')
- Path.resolve(strict=False)¶
Зробіть шлях абсолютним, дозволяючи будь-які символічні посилання. Повертається новий об’єкт шляху:
>>> p = Path() >>> p PosixPath('.') >>> p.resolve() PosixPath('/home/antoine/pathlib')
Компоненти «
..
» також видаляються (це єдиний спосіб зробити це):>>> p = Path('docs/../setup.py') >>> p.resolve() PosixPath('/home/antoine/pathlib/setup.py')
If the path doesn’t exist and strict is
True
,FileNotFoundError
is raised. If strict isFalse
, the path is resolved as far as possible and any remainder is appended without checking whether it exists. If an infinite loop is encountered along the resolution path,RuntimeError
is raised.Змінено в версії 3.6: The strict parameter was added (pre-3.6 behavior is strict).
- Path.readlink()¶
Повертає шлях, на який вказує символічне посилання (як повертає
os.readlink()
):>>> p = Path('mylink') >>> p.symlink_to('setup.py') >>> p.readlink() PosixPath('setup.py')
Added in version 3.9.
Querying file type and status¶
Змінено в версії 3.8: exists()
, is_dir()
, is_file()
,
is_mount()
, is_symlink()
,
is_block_device()
, is_char_device()
,
is_fifo()
, is_socket()
now return False
instead of raising an exception for paths that contain characters
unrepresentable at the OS level.
- Path.stat(*, follow_symlinks=True)¶
Return an
os.stat_result
object containing information about this path, likeos.stat()
. The result is looked up at each call to this method.Цей метод зазвичай слідує за символічними посиланнями; щоб стати символічним посиланням, додайте аргумент
follow_symlinks=False
або використовуйтеlstat()
.>>> p = Path('setup.py') >>> p.stat().st_size 956 >>> p.stat().st_mtime 1327883547.852554
Змінено в версії 3.10: Додано параметр follow_symlinks.
- Path.lstat()¶
Подібно до
Path.stat()
, але якщо шлях вказує на символічне посилання, повертається інформація про символічне посилання, а не про його ціль.
- Path.exists(*, follow_symlinks=True)¶
Return
True
if the path points to an existing file or directory.This method normally follows symlinks; to check if a symlink exists, add the argument
follow_symlinks=False
.>>> Path('.').exists() True >>> Path('setup.py').exists() True >>> Path('/etc').exists() True >>> Path('nonexistentfile').exists() False
Змінено в версії 3.12: Додано параметр follow_symlinks.
- Path.is_file()¶
Return
True
if the path points to a regular file (or a symbolic link pointing to a regular file),False
if it points to another kind of file.False
також повертається, якщо шлях не існує або це несправне символічне посилання; інші помилки (наприклад, помилки дозволу) поширюються.
- Path.is_dir()¶
Return
True
if the path points to a directory (or a symbolic link pointing to a directory),False
if it points to another kind of file.False
також повертається, якщо шлях не існує або це несправне символічне посилання; інші помилки (наприклад, помилки дозволу) поширюються.
- Path.is_symlink()¶
Повертає
True
, якщо шлях вказує на символічне посилання,False
інакше.False
також повертається, якщо шлях не існує; інші помилки (наприклад, помилки дозволу) поширюються.
- Path.is_junction()¶
Return
True
if the path points to a junction, andFalse
for any other type of file. Currently only Windows supports junctions.Added in version 3.12.
- Path.is_mount()¶
Return
True
if the path is a mount point: a point in a file system where a different file system has been mounted. On POSIX, the function checks whether path’s parent,path/..
, is on a different device than path, or whetherpath/..
and path point to the same i-node on the same device — this should detect mount points for all Unix and POSIX variants. On Windows, a mount point is considered to be a drive letter root (e.g.c:\
), a UNC share (e.g.\\server\share
), or a mounted filesystem directory.Added in version 3.7.
Змінено в версії 3.12: Додано підтримку Windows.
- Path.is_socket()¶
Повертає
True
, якщо шлях вказує на сокет Unix (або символічне посилання, що вказує на сокет Unix),False
, якщо він вказує на інший тип файлу.False
також повертається, якщо шлях не існує або це несправне символічне посилання; інші помилки (наприклад, помилки дозволу) поширюються.
- Path.is_fifo()¶
Повертає
True
, якщо шлях вказує на FIFO (або символічне посилання, що вказує на FIFO),False
, якщо він вказує на інший тип файлу.False
також повертається, якщо шлях не існує або це несправне символічне посилання; інші помилки (наприклад, помилки дозволу) поширюються.
- Path.is_block_device()¶
Повертає
True
, якщо шлях вказує на блоковий пристрій (або символічне посилання, що вказує на блоковий пристрій),False
, якщо він вказує на інший тип файлу.False
також повертається, якщо шлях не існує або це несправне символічне посилання; інші помилки (наприклад, помилки дозволу) поширюються.
- Path.is_char_device()¶
Повертає
True
, якщо шлях вказує на символьний пристрій (або символічне посилання, що вказує на символьний пристрій),False
, якщо він вказує на інший тип файлу.False
також повертається, якщо шлях не існує або це несправне символічне посилання; інші помилки (наприклад, помилки дозволу) поширюються.
- Path.samefile(other_path)¶
Повертає, чи цей шлях вказує на той самий файл, що й other_path, який може бути об’єктом Path або рядком. Семантика подібна до
os.path.samefile()
іos.path.samestat()
.OSError
може виникнути, якщо з певної причини неможливо отримати доступ до будь-якого файлу.>>> p = Path('spam') >>> q = Path('eggs') >>> p.samefile(q) False >>> p.samefile('spam') True
Added in version 3.5.
Reading and writing files¶
- Path.open(mode='r', buffering=-1, encoding=None, errors=None, newline=None)¶
Відкрийте файл, на який вказує шлях, як це робить вбудована функція
open()
:>>> p = Path('setup.py') >>> with p.open() as f: ... f.readline() ... '#!/usr/bin/env python3\n'
- Path.read_text(encoding=None, errors=None)¶
Повертає декодований вміст вказаного файлу як рядок:
>>> p = Path('my_text_file') >>> p.write_text('Text file contents') 18 >>> p.read_text() 'Text file contents'
Файл відкривається, а потім закривається. Необов’язкові параметри мають те саме значення, що й у
open()
.Added in version 3.5.
- Path.read_bytes()¶
Повертає двійковий вміст зазначеного файлу як об’єкт bytes:
>>> p = Path('my_binary_file') >>> p.write_bytes(b'Binary file contents') 20 >>> p.read_bytes() b'Binary file contents'
Added in version 3.5.
- Path.write_text(data, encoding=None, errors=None, newline=None)¶
Відкрийте вказаний файл у текстовому режимі, запишіть у нього data та закрийте файл:
>>> p = Path('my_text_file') >>> p.write_text('Text file contents') 18 >>> p.read_text() 'Text file contents'
Існуючий файл із такою ж назвою буде перезаписано. Необов’язкові параметри мають те саме значення, що й у
open()
.Added in version 3.5.
Змінено в версії 3.10: Додано параметр новий рядок.
- Path.write_bytes(data)¶
Відкрийте вказаний файл у байтовому режимі, запишіть у нього data та закрийте файл:
>>> p = Path('my_binary_file') >>> p.write_bytes(b'Binary file contents') 20 >>> p.read_bytes() b'Binary file contents'
Існуючий файл із такою ж назвою буде перезаписано.
Added in version 3.5.
Reading directories¶
- Path.iterdir()¶
Коли шлях вказує на каталог, видає об’єкти шляху вмісту каталогу:
>>> p = Path('docs') >>> for child in p.iterdir(): child ... PosixPath('docs/conf.py') PosixPath('docs/_templates') PosixPath('docs/make.bat') PosixPath('docs/index.rst') PosixPath('docs/_build') PosixPath('docs/_static') PosixPath('docs/Makefile')
The children are yielded in arbitrary order, and the special entries
'.'
and'..'
are not included. If a file is removed from or added to the directory after creating the iterator, it is unspecified whether a path object for that file is included.If the path is not a directory or otherwise inaccessible,
OSError
is raised.
- Path.glob(pattern, *, case_sensitive=None)¶
Помістіть заданий відносний шаблон у каталог, представлений цим шляхом, одержуючи всі відповідні файли (будь-якого типу):
>>> sorted(Path('.').glob('*.py')) [PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')] >>> sorted(Path('.').glob('*/*.py')) [PosixPath('docs/conf.py')]
Patterns are the same as for
fnmatch
, with the addition of «**
» which means «this directory and all subdirectories, recursively». In other words, it enables recursive globbing:>>> sorted(Path('.').glob('**/*.py')) [PosixPath('build/lib/pathlib.py'), PosixPath('docs/conf.py'), PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')]
This method calls
Path.is_dir()
on the top-level directory and propagates anyOSError
exception that is raised. SubsequentOSError
exceptions from scanning directories are suppressed.By default, or when the case_sensitive keyword-only argument is set to
None
, this method matches paths using platform-specific casing rules: typically, case-sensitive on POSIX, and case-insensitive on Windows. Set case_sensitive toTrue
orFalse
to override this behaviour.Примітка
Using the «
**
» pattern in large directory trees may consume an inordinate amount of time.Викликає подію аудиту
pathlib.Path.glob
з аргументамиself
,pattern
.Змінено в версії 3.11: Return only directories if pattern ends with a pathname components separator (
sep
oraltsep
).Змінено в версії 3.12: The case_sensitive parameter was added.
- Path.rglob(pattern, *, case_sensitive=None)¶
Glob the given relative pattern recursively. This is like calling
Path.glob()
with «**/
» added in front of the pattern, where patterns are the same as forfnmatch
:>>> sorted(Path().rglob("*.py")) [PosixPath('build/lib/pathlib.py'), PosixPath('docs/conf.py'), PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')]
By default, or when the case_sensitive keyword-only argument is set to
None
, this method matches paths using platform-specific casing rules: typically, case-sensitive on POSIX, and case-insensitive on Windows. Set case_sensitive toTrue
orFalse
to override this behaviour.Викликає подію аудиту
pathlib.Path.rglob
з аргументамиself
,pattern
.Змінено в версії 3.11: Return only directories if pattern ends with a pathname components separator (
sep
oraltsep
).Змінено в версії 3.12: The case_sensitive parameter was added.
- Path.walk(top_down=True, on_error=None, follow_symlinks=False)¶
Generate the file names in a directory tree by walking the tree either top-down or bottom-up.
For each directory in the directory tree rooted at self (including self but excluding „.“ and „..“), the method yields a 3-tuple of
(dirpath, dirnames, filenames)
.dirpath is a
Path
to the directory currently being walked, dirnames is a list of strings for the names of subdirectories in dirpath (excluding'.'
and'..'
), and filenames is a list of strings for the names of the non-directory files in dirpath. To get a full path (which begins with self) to a file or directory in dirpath, dodirpath / name
. Whether or not the lists are sorted is file system-dependent.If the optional argument top_down is true (which is the default), the triple for a directory is generated before the triples for any of its subdirectories (directories are walked top-down). If top_down is false, the triple for a directory is generated after the triples for all of its subdirectories (directories are walked bottom-up). No matter the value of top_down, the list of subdirectories is retrieved before the triples for the directory and its subdirectories are walked.
When top_down is true, the caller can modify the dirnames list in-place (for example, using
del
or slice assignment), andPath.walk()
will only recurse into the subdirectories whose names remain in dirnames. This can be used to prune the search, or to impose a specific order of visiting, or even to informPath.walk()
about directories the caller creates or renames before it resumesPath.walk()
again. Modifying dirnames when top_down is false has no effect on the behavior ofPath.walk()
since the directories in dirnames have already been generated by the time dirnames is yielded to the caller.By default, errors from
os.scandir()
are ignored. If the optional argument on_error is specified, it should be a callable; it will be called with one argument, anOSError
instance. The callable can handle the error to continue the walk or re-raise it to stop the walk. Note that the filename is available as thefilename
attribute of the exception object.By default,
Path.walk()
does not follow symbolic links, and instead adds them to the filenames list. Set follow_symlinks to true to resolve symlinks and place them in dirnames and filenames as appropriate for their targets, and consequently visit directories pointed to by symlinks (where supported).Примітка
Be aware that setting follow_symlinks to true can lead to infinite recursion if a link points to a parent directory of itself.
Path.walk()
does not keep track of the directories it has already visited.Примітка
Path.walk()
assumes the directories it walks are not modified during execution. For example, if a directory from dirnames has been replaced with a symlink and follow_symlinks is false,Path.walk()
will still try to descend into it. To prevent such behavior, remove directories from dirnames as appropriate.Примітка
Unlike
os.walk()
,Path.walk()
lists symlinks to directories in filenames if follow_symlinks is false.This example displays the number of bytes used by all files in each directory, while ignoring
__pycache__
directories:from pathlib import Path for root, dirs, files in Path("cpython/Lib/concurrent").walk(on_error=print): print( root, "consumes", sum((root / file).stat().st_size for file in files), "bytes in", len(files), "non-directory files" ) if '__pycache__' in dirs: dirs.remove('__pycache__')
This next example is a simple implementation of
shutil.rmtree()
. Walking the tree bottom-up is essential asrmdir()
doesn’t allow deleting a directory before it is empty:# Delete everything reachable from the directory "top". # CAUTION: This is dangerous! For example, if top == Path('/'), # it could delete all of your files. for root, dirs, files in top.walk(top_down=False): for name in files: (root / name).unlink() for name in dirs: (root / name).rmdir()
Added in version 3.12.
Creating files and directories¶
- Path.touch(mode=0o666, exist_ok=True)¶
Create a file at this given path. If mode is given, it is combined with the process’s
umask
value to determine the file mode and access flags. If the file already exists, the function succeeds when exist_ok is true (and its modification time is updated to the current time), otherwiseFileExistsError
is raised.Дивись також
The
open()
,write_text()
andwrite_bytes()
methods are often used to create files.
- Path.mkdir(mode=0o777, parents=False, exist_ok=False)¶
Create a new directory at this given path. If mode is given, it is combined with the process’s
umask
value to determine the file mode and access flags. If the path already exists,FileExistsError
is raised.Якщо parents має значення true, будь-які відсутні батьки цього шляху створюються за потреби; вони створюються з дозволами за замовчуванням без урахування mode (імітація команди POSIX
mkdir -p
).Якщо parents має значення false (за замовчуванням), відсутній батьківський елемент викликає
FileNotFoundError
.Якщо exist_ok має значення false (за замовчуванням),
FileExistsError
викликається, якщо цільовий каталог уже існує.If exist_ok is true,
FileExistsError
will not be raised unless the given path already exists in the file system and is not a directory (same behavior as the POSIXmkdir -p
command).Змінено в версії 3.5: Додано параметр exist_ok.
- Path.symlink_to(target, target_is_directory=False)¶
Make this path a symbolic link pointing to target.
On Windows, a symlink represents either a file or a directory, and does not morph to the target dynamically. If the target is present, the type of the symlink will be created to match. Otherwise, the symlink will be created as a directory if target_is_directory is true or a file symlink (the default) otherwise. On non-Windows platforms, target_is_directory is ignored.
>>> p = Path('mylink') >>> p.symlink_to('setup.py') >>> p.resolve() PosixPath('/home/antoine/pathlib/setup.py') >>> p.stat().st_size 956 >>> p.lstat().st_size 8
Примітка
Порядок аргументів (посилання, ціль) є зворотним порядку
os.symlink()
.
Renaming and deleting¶
- Path.rename(target)¶
Rename this file or directory to the given target, and return a new
Path
instance pointing to target. On Unix, if target exists and is a file, it will be replaced silently if the user has permission. On Windows, if target exists,FileExistsError
will be raised. target can be either a string or another path object:>>> p = Path('foo') >>> p.open('w').write('some text') 9 >>> target = Path('bar') >>> p.rename(target) PosixPath('bar') >>> target.open().read() 'some text'
The target path may be absolute or relative. Relative paths are interpreted relative to the current working directory, not the directory of the
Path
object.It is implemented in terms of
os.rename()
and gives the same guarantees.Змінено в версії 3.8: Added return value, return the new
Path
instance.
- Path.replace(target)¶
Rename this file or directory to the given target, and return a new
Path
instance pointing to target. If target points to an existing file or empty directory, it will be unconditionally replaced.The target path may be absolute or relative. Relative paths are interpreted relative to the current working directory, not the directory of the
Path
object.Змінено в версії 3.8: Added return value, return the new
Path
instance.
- Path.unlink(missing_ok=False)¶
Видаліть цей файл або символічне посилання. Якщо шлях вказує на каталог, замість цього використовуйте
Path.rmdir()
.Якщо missing_ok має значення false (за замовчуванням),
FileNotFoundError
викликається, якщо шлях не існує.Якщо missing_ok має значення true, винятки
FileNotFoundError
ігноруватимуться (така ж поведінка, що й команда POSIXrm -f
).Змінено в версії 3.8: Додано параметр missing_ok.
- Path.rmdir()¶
Видаліть цей каталог. Каталог має бути порожнім.
Permissions and ownership¶
- Path.owner()¶
Return the name of the user owning the file.
KeyError
is raised if the file’s user identifier (UID) isn’t found in the system database.
- Path.group()¶
Return the name of the group owning the file.
KeyError
is raised if the file’s group identifier (GID) isn’t found in the system database.
- Path.chmod(mode, *, follow_symlinks=True)¶
Змініть режим файлу та дозволи, наприклад
os.chmod()
.Цей метод зазвичай слідує за символічними посиланнями. Деякі різновиди Unix підтримують зміну дозволів для самого символічного посилання; на цих платформах ви можете додати аргумент
follow_symlinks=False
або використовуватиlchmod()
.>>> p = Path('setup.py') >>> p.stat().st_mode 33277 >>> p.chmod(0o444) >>> p.stat().st_mode 33060
Змінено в версії 3.10: Додано параметр follow_symlinks.
- Path.lchmod(mode)¶
Подібно до
Path.chmod()
, але якщо шлях вказує на символічне посилання, змінюється режим символічного посилання, а не його призначення.
Correspondence to tools in the os
module¶
Нижче наведено таблицю, що відображає різні функції os
на їхні відповідні еквіваленти PurePath
/Path
.
|
|
|
|
|
|
|
|
|
|
Примітки