pathlib
— 객체 지향 파일 시스템 경로¶
버전 3.4에 추가.
소스 코드: Lib/pathlib.py
이 모듈은 다른 운영 체제에 적합한 의미 체계를 가진 파일 시스템 경로를 나타내는 클래스를 제공합니다. 경로 클래스는 I/O 없이 순수한 계산 연산을 제공하는 순수한 경로와 순수한 경로를 상속하지만, I/O 연산도 제공하는 구상 경로로 구분됩니다.
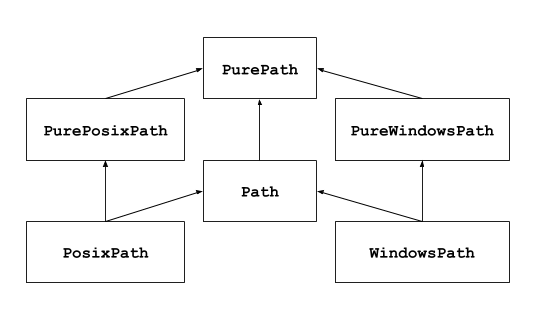
이전에 이 모듈을 사용한 적이 없거나 어떤 클래스가 작업에 적합한지 확신이 없다면, Path
가 가장 적합할 가능성이 높습니다. 코드가 실행되는 플랫폼의 구상 경로를 인스턴스화 합니다.
순수한 경로는 특별한 경우에 유용합니다; 예를 들면:
유닉스 기계에서 윈도우 경로를 조작하려고 할 때 (또는 그 반대). 유닉스에서 실행할 때는
WindowsPath
를 인스턴스화 할 수 없지만,PureWindowsPath
는 인스턴스화 할 수 있습니다.코드가 실제로 OS에 액세스하지 않고 경로만 조작한다는 확신이 필요할 때. 이 경우, 순수 클래스 중 하나를 인스턴스화 하면 OS 액세스 연산이 없어서 유용 할 수 있습니다.
더 보기
PEP 428: pathlib 모듈 – 객체 지향 파일 시스템 경로.
더 보기
문자열에 대한 저수준 경로 조작을 위해, os.path
모듈을 사용할 수도 있습니다.
기본 사용¶
메인 클래스 임포트 하기:
>>> from pathlib import Path
서브 디렉터리 나열하기:
>>> p = Path('.')
>>> [x for x in p.iterdir() if x.is_dir()]
[PosixPath('.hg'), PosixPath('docs'), PosixPath('dist'),
PosixPath('__pycache__'), PosixPath('build')]
이 디렉터리 트리에 있는 파이썬 소스 파일 나열하기:
>>> list(p.glob('**/*.py'))
[PosixPath('test_pathlib.py'), PosixPath('setup.py'),
PosixPath('pathlib.py'), PosixPath('docs/conf.py'),
PosixPath('build/lib/pathlib.py')]
디렉터리 트리 내에서 탐색하기:
>>> p = Path('/etc')
>>> q = p / 'init.d' / 'reboot'
>>> q
PosixPath('/etc/init.d/reboot')
>>> q.resolve()
PosixPath('/etc/rc.d/init.d/halt')
경로 속성 조회하기:
>>> q.exists()
True
>>> q.is_dir()
False
파일 열기:
>>> with q.open() as f: f.readline()
...
'#!/bin/bash\n'
순수한 경로¶
순수한 경로 객체는 실제로 파일 시스템에 액세스하지 않는 경로 처리 연산을 제공합니다. 이 클래스에 액세스하는 방법에는 세 가지가 있으며, 플레이버(flavours)라고도 부릅니다:
-
class
pathlib.
PurePath
(*pathsegments)¶ 시스템의 경로 플레이버를 나타내는 일반 클래스 (인스턴스화 하면
PurePosixPath
나PureWindowsPath
를 만듭니다):>>> PurePath('setup.py') # Running on a Unix machine PurePosixPath('setup.py')
pathsegments의 각 요소는 경로 세그먼트를 나타내는 문자열, 문자열을 반환하는
os.PathLike
인터페이스를 구현하는 객체 또는 다른 경로 객체일 수 있습니다:>>> PurePath('foo', 'some/path', 'bar') PurePosixPath('foo/some/path/bar') >>> PurePath(Path('foo'), Path('bar')) PurePosixPath('foo/bar')
pathsegments가 비어 있으면, 현재 디렉터리를 가정합니다:
>>> PurePath() PurePosixPath('.')
If a segment is an absolute path, all previous segments are ignored (like
os.path.join()
):>>> PurePath('/etc', '/usr', 'lib64') PurePosixPath('/usr/lib64') >>> PureWindowsPath('c:/Windows', 'd:bar') PureWindowsPath('d:bar')
On Windows, the drive is not reset when a rooted relative path segment (e.g.,
r'\foo'
) is encountered:>>> PureWindowsPath('c:/Windows', '/Program Files') PureWindowsPath('c:/Program Files')
Spurious slashes and single dots are collapsed, but double dots (
'..'
) and leading double slashes ('//'
) are not, since this would change the meaning of a path for various reasons (e.g. symbolic links, UNC paths):>>> PurePath('foo//bar') PurePosixPath('foo/bar') >>> PurePath('//foo/bar') PurePosixPath('//foo/bar') >>> PurePath('foo/./bar') PurePosixPath('foo/bar') >>> PurePath('foo/../bar') PurePosixPath('foo/../bar')
(나이브한 접근법은
PurePosixPath('foo/../bar')
를PurePosixPath('bar')
와 동등하게 만드는데,foo
가 다른 디렉터리에 대한 심볼릭 링크일 때는 잘못됩니다)순수한 경로 객체는
os.PathLike
인터페이스를 구현하여, 이 인터페이스가 허용되는 모든 위치에서 사용할 수 있습니다.버전 3.6에서 변경:
os.PathLike
인터페이스에 대한 지원이 추가되었습니다.
-
class
pathlib.
PurePosixPath
(*pathsegments)¶ PurePath
의 서브 클래스, 이 경로 플레이버는 윈도우 이외의 파일 시스템 경로를 나타냅니다:>>> PurePosixPath('/etc') PurePosixPath('/etc')
pathsegments는
PurePath
와 유사하게 지정됩니다.
-
class
pathlib.
PureWindowsPath
(*pathsegments)¶ A subclass of
PurePath
, this path flavour represents Windows filesystem paths, including UNC paths:>>> PureWindowsPath('c:/Program Files/') PureWindowsPath('c:/Program Files') >>> PureWindowsPath('//server/share/file') PureWindowsPath('//server/share/file')
pathsegments는
PurePath
와 유사하게 지정됩니다.
실행 중인 시스템과 관계없이, 이러한 모든 클래스를 인스턴스화 할 수 있는데, 시스템 호출을 수행하는 연산을 제공하지 않기 때문입니다.
일반 속성¶
Paths are immutable and hashable. Paths of a same flavour are comparable and orderable. These properties respect the flavour’s case-folding semantics:
>>> PurePosixPath('foo') == PurePosixPath('FOO')
False
>>> PureWindowsPath('foo') == PureWindowsPath('FOO')
True
>>> PureWindowsPath('FOO') in { PureWindowsPath('foo') }
True
>>> PureWindowsPath('C:') < PureWindowsPath('d:')
True
다른 플레이버의 경로는 다르다고 비교되며 대소 비교할 수 없습니다:
>>> PureWindowsPath('foo') == PurePosixPath('foo')
False
>>> PureWindowsPath('foo') < PurePosixPath('foo')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: '<' not supported between instances of 'PureWindowsPath' and 'PurePosixPath'
연산자¶
The slash operator helps create child paths, like os.path.join()
.
If the argument is an absolute path, the previous path is ignored.
On Windows, the drive is not reset when the argument is a rooted
relative path (e.g., r'\foo'
):
>>> p = PurePath('/etc')
>>> p
PurePosixPath('/etc')
>>> p / 'init.d' / 'apache2'
PurePosixPath('/etc/init.d/apache2')
>>> q = PurePath('bin')
>>> '/usr' / q
PurePosixPath('/usr/bin')
>>> p / '/an_absolute_path'
PurePosixPath('/an_absolute_path')
>>> PureWindowsPath('c:/Windows', '/Program Files')
PureWindowsPath('c:/Program Files')
경로 객체는 os.PathLike
을 구현하는 객체가 허용되는 모든 곳에서 사용할 수 있습니다:
>>> import os
>>> p = PurePath('/etc')
>>> os.fspath(p)
'/etc'
경로의 문자열 표현은 원시 파일 시스템 경로 자체(네이티브 형식으로, 예를 들어 윈도우에서 역 슬래시)로, 파일 경로를 문자열로 받아들이는 모든 함수에 전달할 수 있습니다:
>>> p = PurePath('/etc')
>>> str(p)
'/etc'
>>> p = PureWindowsPath('c:/Program Files')
>>> str(p)
'c:\\Program Files'
마찬가지로, 경로에 대해 bytes
를 호출하면 os.fsencode()
로 인코딩된 바이트열 객체로 원시 파일 시스템 경로를 제공합니다:
>>> bytes(p)
b'/etc'
참고
bytes
호출은 유닉스에서만 권장됩니다. 윈도우에서, 유니코드 형식이 파일 시스템 경로의 규범적(canonical) 표현입니다.
개별 부분에 액세스하기¶
경로의 개별 “부분”(구성 요소)에 액세스하려면, 다음 프로퍼티를 사용하십시오:
-
PurePath.
parts
¶ 경로의 다양한 구성 요소로의 액세스를 제공하는 튜플:
>>> p = PurePath('/usr/bin/python3') >>> p.parts ('/', 'usr', 'bin', 'python3') >>> p = PureWindowsPath('c:/Program Files/PSF') >>> p.parts ('c:\\', 'Program Files', 'PSF')
(드라이브와 로컬 루트가 단일 부분으로 다시 그룹화되는 방식에 유의하십시오)
메서드와 프로퍼티¶
순수한 경로는 다음과 같은 메서드와 프로퍼티를 제공합니다:
-
PurePath.
drive
¶ 드라이브 문자나 이름을 나타내는 문자열, 있다면:
>>> PureWindowsPath('c:/Program Files/').drive 'c:' >>> PureWindowsPath('/Program Files/').drive '' >>> PurePosixPath('/etc').drive ''
UNC 공유도 드라이브로 간주합니다:
>>> PureWindowsPath('//host/share/foo.txt').drive '\\\\host\\share'
-
PurePath.
root
¶ (로컬이나 글로벌) 루트를 나타내는 문자열, 있다면:
>>> PureWindowsPath('c:/Program Files/').root '\\' >>> PureWindowsPath('c:Program Files/').root '' >>> PurePosixPath('/etc').root '/'
UNC 공유에는 항상 루트가 있습니다:
>>> PureWindowsPath('//host/share').root '\\'
If the path starts with more than two successive slashes,
PurePosixPath
collapses them:>>> PurePosixPath('//etc').root '//' >>> PurePosixPath('///etc').root '/' >>> PurePosixPath('////etc').root '/'
참고
This behavior conforms to The Open Group Base Specifications Issue 6, paragraph 4.11 Pathname Resolution:
“A pathname that begins with two successive slashes may be interpreted in an implementation-defined manner, although more than two leading slashes shall be treated as a single slash.”
-
PurePath.
anchor
¶ 드라이브와 루트의 이어 붙이기:
>>> PureWindowsPath('c:/Program Files/').anchor 'c:\\' >>> PureWindowsPath('c:Program Files/').anchor 'c:' >>> PurePosixPath('/etc').anchor '/' >>> PureWindowsPath('//host/share').anchor '\\\\host\\share\\'
-
PurePath.
parents
¶ 경로의 논리적 조상에 대한 액세스를 제공하는 불변 시퀀스:
>>> p = PureWindowsPath('c:/foo/bar/setup.py') >>> p.parents[0] PureWindowsPath('c:/foo/bar') >>> p.parents[1] PureWindowsPath('c:/foo') >>> p.parents[2] PureWindowsPath('c:/')
버전 3.10에서 변경: The parents sequence now supports slices and negative index values.
-
PurePath.
parent
¶ 경로의 논리적 부모:
>>> p = PurePosixPath('/a/b/c/d') >>> p.parent PurePosixPath('/a/b/c')
앵커나 빈 경로를 넘어갈 수 없습니다:
>>> p = PurePosixPath('/') >>> p.parent PurePosixPath('/') >>> p = PurePosixPath('.') >>> p.parent PurePosixPath('.')
참고
이것은 순수한 어휘(lexical) 연산이라서, 다음과 같이 동작합니다:
>>> p = PurePosixPath('foo/..') >>> p.parent PurePosixPath('foo')
임의의 파일 시스템 경로를 위쪽으로 걸어가려면, 먼저
Path.resolve()
를 호출해서 심볼릭 링크를 결정하고 “..” 구성 요소를 제거하는 것이 좋습니다.
-
PurePath.
name
¶ 드라이브와 루트를 제외하고, 마지막 경로 구성 요소를 나타내는 문자열, 있다면:
>>> PurePosixPath('my/library/setup.py').name 'setup.py'
UNC 드라이브 이름은 고려되지 않습니다:
>>> PureWindowsPath('//some/share/setup.py').name 'setup.py' >>> PureWindowsPath('//some/share').name ''
-
PurePath.
suffix
¶ 마지막 구성 요소의 파일 확장자, 있다면:
>>> PurePosixPath('my/library/setup.py').suffix '.py' >>> PurePosixPath('my/library.tar.gz').suffix '.gz' >>> PurePosixPath('my/library').suffix ''
-
PurePath.
suffixes
¶ 경로의 파일 확장자 리스트:
>>> PurePosixPath('my/library.tar.gar').suffixes ['.tar', '.gar'] >>> PurePosixPath('my/library.tar.gz').suffixes ['.tar', '.gz'] >>> PurePosixPath('my/library').suffixes []
-
PurePath.
stem
¶ suffix가 없는, 마지막 경로 구성 요소:
>>> PurePosixPath('my/library.tar.gz').stem 'library.tar' >>> PurePosixPath('my/library.tar').stem 'library' >>> PurePosixPath('my/library').stem 'library'
-
PurePath.
as_posix
()¶ 슬래시(
/
)가 있는 경로의 문자열 표현을 반환합니다:>>> p = PureWindowsPath('c:\\windows') >>> str(p) 'c:\\windows' >>> p.as_posix() 'c:/windows'
-
PurePath.
as_uri
()¶ 경로를
file
URI로 나타냅니다. 경로가 절대적이지 않으면ValueError
가 발생합니다.>>> p = PurePosixPath('/etc/passwd') >>> p.as_uri() 'file:///etc/passwd' >>> p = PureWindowsPath('c:/Windows') >>> p.as_uri() 'file:///c:/Windows'
-
PurePath.
is_absolute
()¶ 경로가 절대적인지 아닌지를 반환합니다. 루트와 (플레이버가 허락하면) 드라이브가 모두 있으면 경로를 절대적이라고 간주합니다:
>>> PurePosixPath('/a/b').is_absolute() True >>> PurePosixPath('a/b').is_absolute() False >>> PureWindowsPath('c:/a/b').is_absolute() True >>> PureWindowsPath('/a/b').is_absolute() False >>> PureWindowsPath('c:').is_absolute() False >>> PureWindowsPath('//some/share').is_absolute() True
-
PurePath.
is_relative_to
(*other)¶ 이 경로가 other 경로에 상대적인지를 반환합니다.
>>> p = PurePath('/etc/passwd') >>> p.is_relative_to('/etc') True >>> p.is_relative_to('/usr') False
버전 3.9에 추가.
-
PurePath.
is_reserved
()¶ PureWindowsPath
에서는, 경로를 윈도우에서 예약된 것으로 간주하면True
를, 그렇지 않으면False
를 반환합니다.PurePosixPath
에서는, 항상False
가 반환됩니다.>>> PureWindowsPath('nul').is_reserved() True >>> PurePosixPath('nul').is_reserved() False
예약된 경로에 대한 파일 시스템 호출은 실마리 없이 실패하거나 의도하지 않은 결과를 초래할 수 있습니다.
-
PurePath.
joinpath
(*other)¶ 이 메서드를 호출하는 것은 경로를 각 other 인자와 차례로 결합하는 것과 동등합니다:
>>> PurePosixPath('/etc').joinpath('passwd') PurePosixPath('/etc/passwd') >>> PurePosixPath('/etc').joinpath(PurePosixPath('passwd')) PurePosixPath('/etc/passwd') >>> PurePosixPath('/etc').joinpath('init.d', 'apache2') PurePosixPath('/etc/init.d/apache2') >>> PureWindowsPath('c:').joinpath('/Program Files') PureWindowsPath('c:/Program Files')
-
PurePath.
match
(pattern)¶ 이 경로를 제공된 glob 스타일 패턴과 일치시킵니다. 일치하면
True
를, 그렇지 않으면False
를 반환합니다.pattern이 상대적이면, 경로는 상대적이거나 절대적일 수 있으며, 일치는 오른쪽으로부터 수행됩니다:
>>> PurePath('a/b.py').match('*.py') True >>> PurePath('/a/b/c.py').match('b/*.py') True >>> PurePath('/a/b/c.py').match('a/*.py') False
pattern이 절대적이면, 경로는 절대적이어야 하고, 전체 경로가 일치해야 합니다:
>>> PurePath('/a.py').match('/*.py') True >>> PurePath('a/b.py').match('/*.py') False
다른 메서드와 마찬가지로, 대소 문자를 구분할지는 플랫폼 기본값을 따릅니다:
>>> PurePosixPath('b.py').match('*.PY') False >>> PureWindowsPath('b.py').match('*.PY') True
-
PurePath.
relative_to
(*other)¶ 이 경로의 other로 표시되는 경로에 상대적인 버전을 계산합니다. 불가능하면 ValueError가 발생합니다:
>>> p = PurePosixPath('/etc/passwd') >>> p.relative_to('/') PurePosixPath('etc/passwd') >>> p.relative_to('/etc') PurePosixPath('passwd') >>> p.relative_to('/usr') Traceback (most recent call last): File "<stdin>", line 1, in <module> File "pathlib.py", line 694, in relative_to .format(str(self), str(formatted))) ValueError: '/etc/passwd' is not in the subpath of '/usr' OR one path is relative and the other absolute.
참고: 이 함수는
PurePath
의 일부이며 문자열과 함께 작동합니다. 하부 파일 구조를 확인하거나 액세스하지 않습니다.
-
PurePath.
with_name
(name)¶ name
이 변경된 새 경로를 반환합니다. 원래 경로에 이름(name)이 없으면 ValueError가 발생합니다:>>> p = PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>> p.with_name('setup.py') PureWindowsPath('c:/Downloads/setup.py') >>> p = PureWindowsPath('c:/') >>> p.with_name('setup.py') Traceback (most recent call last): File "<stdin>", line 1, in <module> File "/home/antoine/cpython/default/Lib/pathlib.py", line 751, in with_name raise ValueError("%r has an empty name" % (self,)) ValueError: PureWindowsPath('c:/') has an empty name
-
PurePath.
with_stem
(stem)¶ stem
이 변경된 새 경로를 반환합니다. 원래 경로에 이름(name)이 없으면, ValueError가 발생합니다:>>> p = PureWindowsPath('c:/Downloads/draft.txt') >>> p.with_stem('final') PureWindowsPath('c:/Downloads/final.txt') >>> p = PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>> p.with_stem('lib') PureWindowsPath('c:/Downloads/lib.gz') >>> p = PureWindowsPath('c:/') >>> p.with_stem('') Traceback (most recent call last): File "<stdin>", line 1, in <module> File "/home/antoine/cpython/default/Lib/pathlib.py", line 861, in with_stem return self.with_name(stem + self.suffix) File "/home/antoine/cpython/default/Lib/pathlib.py", line 851, in with_name raise ValueError("%r has an empty name" % (self,)) ValueError: PureWindowsPath('c:/') has an empty name
버전 3.9에 추가.
-
PurePath.
with_suffix
(suffix)¶ suffix
가 변경된 새 경로를 반환합니다. 원래 경로에 접미사(suffix)가 없으면, 새 suffix가 대신 추가됩니다. suffix가 빈 문자열이면, 원래 접미사가 제거됩니다:>>> p = PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>> p.with_suffix('.bz2') PureWindowsPath('c:/Downloads/pathlib.tar.bz2') >>> p = PureWindowsPath('README') >>> p.with_suffix('.txt') PureWindowsPath('README.txt') >>> p = PureWindowsPath('README.txt') >>> p.with_suffix('') PureWindowsPath('README')
구상 경로¶
구상 경로는 순수한 경로 클래스의 서브 클래스입니다. 후자가 제공하는 연산 외에도, 경로 객체에 대해 시스템 호출을 수행하는 메서드도 제공합니다. 구상 경로를 인스턴스화 하는 세 가지 방법이 있습니다:
-
class
pathlib.
Path
(*pathsegments)¶ PurePath
의 서브 클래스, 이 클래스는 시스템의 경로 플레이버의 구상 경로를 나타냅니다 (인스턴스화 하면PosixPath
나WindowsPath
를 만듭니다):>>> Path('setup.py') PosixPath('setup.py')
pathsegments는
PurePath
와 유사하게 지정됩니다.
-
class
pathlib.
PosixPath
(*pathsegments)¶ Path
와PurePosixPath
의 서브 클래스, 이 클래스는 윈도우 이외의 구상 파일 시스템 경로를 나타냅니다:>>> PosixPath('/etc') PosixPath('/etc')
pathsegments는
PurePath
와 유사하게 지정됩니다.
-
class
pathlib.
WindowsPath
(*pathsegments)¶ Path
와PureWindowsPath
의 서브 클래스, 이 클래스는 구상 윈도우 파일 시스템 경로를 나타냅니다:>>> WindowsPath('c:/Program Files/') WindowsPath('c:/Program Files')
pathsegments는
PurePath
와 유사하게 지정됩니다.
여러분의 시스템에 해당하는 클래스 플레이버만 인스턴스화 할 수 있습니다 (호환되지 않는 경로 플레이버에 대한 시스템 호출을 허용하면 응용 프로그램에서 버그나 실패가 발생할 수 있습니다):
>>> import os
>>> os.name
'posix'
>>> Path('setup.py')
PosixPath('setup.py')
>>> PosixPath('setup.py')
PosixPath('setup.py')
>>> WindowsPath('setup.py')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "pathlib.py", line 798, in __new__
% (cls.__name__,))
NotImplementedError: cannot instantiate 'WindowsPath' on your system
메서드¶
구상 경로는 순수한 경로 메서드 외에도 다음과 같은 메서드를 제공합니다. 이 메서드 중 많은 것들이 시스템 호출이 실패할 때 (예를 들어 경로가 존재하지 않아서) OSError
를 발생시킬 수 있습니다.
버전 3.8에서 변경: exists()
, is_dir()
, is_file()
, is_mount()
, is_symlink()
, is_block_device()
, is_char_device()
, is_fifo()
, is_socket()
은 이제 OS 수준에서 표현할 수 없는 문자가 포함된 경로에 대해 예외를 발생시키는 대신 False
를 반환합니다.
-
classmethod
Path.
cwd
()¶ 현재 디렉터리를 나타내는 새 경로 객체를 반환합니다.
os.getcwd()
가 반환하는 것과 유사합니다:>>> Path.cwd() PosixPath('/home/antoine/pathlib')
-
classmethod
Path.
home
()¶ Return a new path object representing the user’s home directory (as returned by
os.path.expanduser()
with~
construct). If the home directory can’t be resolved,RuntimeError
is raised.>>> Path.home() PosixPath('/home/antoine')
버전 3.5에 추가.
-
Path.
stat
(*, follow_symlinks=True)¶ os.stat()
과 유사하게, 이 경로에 대한 정보를 포함하는os.stat_result
객체를 반환합니다. 결과는 이 메서드를 호출할 때마다 조회됩니다.This method normally follows symlinks; to stat a symlink add the argument
follow_symlinks=False
, or uselstat()
.>>> p = Path('setup.py') >>> p.stat().st_size 956 >>> p.stat().st_mtime 1327883547.852554
버전 3.10에서 변경: The follow_symlinks parameter was added.
-
Path.
chmod
(mode, *, follow_symlinks=True)¶ Change the file mode and permissions, like
os.chmod()
.This method normally follows symlinks. Some Unix flavours support changing permissions on the symlink itself; on these platforms you may add the argument
follow_symlinks=False
, or uselchmod()
.>>> p = Path('setup.py') >>> p.stat().st_mode 33277 >>> p.chmod(0o444) >>> p.stat().st_mode 33060
버전 3.10에서 변경: The follow_symlinks parameter was added.
-
Path.
exists
()¶ 경로가 기존 파일이나 디렉터리를 가리키는지 여부:
>>> Path('.').exists() True >>> Path('setup.py').exists() True >>> Path('/etc').exists() True >>> Path('nonexistentfile').exists() False
참고
경로가 심볼릭 링크를 가리키면,
exists()
는 심볼릭 링크가 기존 파일이나 디렉터리를 가리키는지를 반환합니다.
-
Path.
expanduser
()¶ Return a new path with expanded
~
and~user
constructs, as returned byos.path.expanduser()
. If a home directory can’t be resolved,RuntimeError
is raised.>>> p = PosixPath('~/films/Monty Python') >>> p.expanduser() PosixPath('/home/eric/films/Monty Python')
버전 3.5에 추가.
-
Path.
glob
(pattern)¶ 이 경로로 표현되는 디렉터리에서, 주어진 상대 pattern을 glob 하여, 일치하는 모든 파일을 (종류와 관계없이) 산출합니다:
>>> sorted(Path('.').glob('*.py')) [PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')] >>> sorted(Path('.').glob('*/*.py')) [PosixPath('docs/conf.py')]
Patterns are the same as for
fnmatch
, with the addition of “**
” which means “this directory and all subdirectories, recursively”. In other words, it enables recursive globbing:>>> sorted(Path('.').glob('**/*.py')) [PosixPath('build/lib/pathlib.py'), PosixPath('docs/conf.py'), PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')]
참고
큰 디렉터리 트리에서 “
**
” 패턴을 사용하면 시간이 오래 걸릴 수 있습니다.인자
self
,pattern
으로 감사 이벤트pathlib.Path.glob
을 발생시킵니다.
-
Path.
is_dir
()¶ 경로가 디렉터리(또는 디렉터리를 가리키는 심볼릭 링크)를 가리키면
True
를 반환하고, 다른 유형의 파일을 가리키면False
를 반환합니다.경로가 존재하지 않거나 깨진 심볼릭 링크일 때도
False
가 반환됩니다; 다른 에러(가령 권한 에러)는 전파됩니다.
-
Path.
is_file
()¶ 경로가 일반 파일(또는 일반 파일을 가리키는 심볼릭 링크)을 가리키면
True
를, 다른 유형의 파일을 가리키면False
를 반환합니다.경로가 존재하지 않거나 깨진 심볼릭 링크일 때도
False
가 반환됩니다; 다른 에러(가령 권한 에러)는 전파됩니다.
-
Path.
is_mount
()¶ 경로가 마운트 지점(mount point)이면
True
를 반환합니다. 마운트 지점은 다른 파일 시스템이 마운트된 파일 시스템의 지점입니다. POSIX에서, 이 함수는 path의 부모path/..
가 path와 다른 장치에 있는지, 또는path/..
와 path가 같은 장치에서 같은 i-노드를 가리키는지를 확인합니다 — 모든 유닉스와 POSIX 변형에서 마운트 지점을 감지해야 합니다. 윈도우에서는 구현되지 않습니다.버전 3.7에 추가.
-
Path.
is_symlink
()¶ 경로가 심볼릭 링크를 가리키면
True
를, 그렇지 않으면False
를 반환합니다.경로가 존재하지 않아도
False
가 반환됩니다; 다른 에러(가령 권한 오류)는 전파됩니다.
-
Path.
is_socket
()¶ 경로가 유닉스 소켓(또는 유닉스 소켓을 가리키는 심볼릭 링크)을 가리키면
True
를, 다른 유형의 파일을 가리키면False
를 반환합니다.경로가 존재하지 않거나 깨진 심볼릭 링크일 때도
False
가 반환됩니다; 다른 에러(가령 권한 에러)는 전파됩니다.
-
Path.
is_fifo
()¶ 경로가 FIFO(또는 FIFO를 가리키는 심볼릭 링크)를 가리키면
True
를, 다른 유형의 파일을 가리키면False
를 반환합니다.경로가 존재하지 않거나 깨진 심볼릭 링크일 때도
False
가 반환됩니다; 다른 에러(가령 권한 에러)는 전파됩니다.
-
Path.
is_block_device
()¶ 경로가 블록 장치(또는 블록 장치를 가리키는 심볼릭 링크)를 가리키면
True
를, 다른 유형의 파일을 가리키면False
를 반환합니다.경로가 존재하지 않거나 깨진 심볼릭 링크일 때도
False
가 반환됩니다; 다른 에러(가령 권한 에러)는 전파됩니다.
-
Path.
is_char_device
()¶ 경로가 문자 장치(또는 문자 장치를 가리키는 심볼릭 링크)를 가리키면
True
를, 다른 유형의 파일을 가리키면False
를 반환합니다.경로가 존재하지 않거나 깨진 심볼릭 링크일 때도
False
가 반환됩니다; 다른 에러(가령 권한 에러)는 전파됩니다.
-
Path.
iterdir
()¶ 경로가 디렉터리를 가리킬 때, 디렉터리 내용의 경로 객체를 산출합니다:
>>> p = Path('docs') >>> for child in p.iterdir(): child ... PosixPath('docs/conf.py') PosixPath('docs/_templates') PosixPath('docs/make.bat') PosixPath('docs/index.rst') PosixPath('docs/_build') PosixPath('docs/_static') PosixPath('docs/Makefile')
The children are yielded in arbitrary order, and the special entries
'.'
and'..'
are not included. If a file is removed from or added to the directory after creating the iterator, whether a path object for that file be included is unspecified.
-
Path.
lchmod
(mode)¶ Path.chmod()
와 비슷하지만, 경로가 심볼릭 링크를 가리키면, 대상이 아닌 심볼릭 링크의 모드가 변경됩니다.
-
Path.
lstat
()¶ Path.stat()
과 비슷하지만, 경로가 심볼릭 링크를 가리키면, 대상이 아닌 심볼릭 링크의 정보를 반환합니다.
-
Path.
mkdir
(mode=0o777, parents=False, exist_ok=False)¶ 이 지정된 경로에 새 디렉터리를 만듭니다. mode가 제공되면, 프로세스의
umask
값과 결합하여 파일 모드와 액세스 플래그를 결정합니다. 경로가 이미 존재하면,FileExistsError
가 발생합니다.parents가 참이면, 이 경로의 누락 된 부모를 필요하면 만듭니다; 이것들은 mode를 고려하지 않고 기본 권한으로 만들어집니다 (POSIX
mkdir -p
명령을 모방합니다).parents가 거짓(기본값)이면, 누락된 부모가
FileNotFoundError
를 발생시킵니다.exist_ok가 거짓(기본값)이면, 대상 디렉터리가 이미 존재하면
FileExistsError
가 발생합니다.exist_ok가 참이면,
FileExistsError
예외가 무시되는데 (POSIXmkdir -p
명령과 같은 동작), 마지막 경로 구성 요소가 이미 존재하는 비 디렉터리 파일이 아닐 때만 그렇습니다.버전 3.5에서 변경: exist_ok 매개 변수가 추가되었습니다.
-
Path.
open
(mode='r', buffering=- 1, encoding=None, errors=None, newline=None)¶ 내장
open()
함수처럼, 경로가 가리키는 파일을 엽니다:>>> p = Path('setup.py') >>> with p.open() as f: ... f.readline() ... '#!/usr/bin/env python3\n'
-
Path.
read_bytes
()¶ 가리키는 파일의 바이너리 내용을 바이트열 객체로 반환합니다:
>>> p = Path('my_binary_file') >>> p.write_bytes(b'Binary file contents') 20 >>> p.read_bytes() b'Binary file contents'
버전 3.5에 추가.
-
Path.
read_text
(encoding=None, errors=None)¶ 가리키는 파일의 디코딩된 내용을 문자열로 반환합니다:
>>> p = Path('my_text_file') >>> p.write_text('Text file contents') 18 >>> p.read_text() 'Text file contents'
파일이 열린 다음에 닫힙니다. 선택적 매개 변수는
open()
과 같은 의미입니다.버전 3.5에 추가.
-
Path.
readlink
()¶ 심볼릭 링크가 가리키는 경로를 반환합니다 (
os.readlink()
가 반환하는 것과 유사합니다):>>> p = Path('mylink') >>> p.symlink_to('setup.py') >>> p.readlink() PosixPath('setup.py')
버전 3.9에 추가.
-
Path.
rename
(target)¶ Rename this file or directory to the given target, and return a new Path instance pointing to target. On Unix, if target exists and is a file, it will be replaced silently if the user has permission. On Windows, if target exists,
FileExistsError
will be raised. target can be either a string or another path object:>>> p = Path('foo') >>> p.open('w').write('some text') 9 >>> target = Path('bar') >>> p.rename(target) PosixPath('bar') >>> target.open().read() 'some text'
target 경로는 절대나 상대 경로일 수 있습니다. 상대 경로는 Path 객체의 디렉터리가 아니라, 현재 작업 디렉터리를 기준으로 해석됩니다.
It is implemented in terms of
os.rename()
and gives the same guarantees.버전 3.8에서 변경: 반환 값을 추가했습니다. 새 Path 인스턴스를 반환합니다.
-
Path.
replace
(target)¶ Rename this file or directory to the given target, and return a new Path instance pointing to target. If target points to an existing file or empty directory, it will be unconditionally replaced.
target 경로는 절대나 상대 경로일 수 있습니다. 상대 경로는 Path 객체의 디렉터리가 아니라, 현재 작업 디렉터리를 기준으로 해석됩니다.
버전 3.8에서 변경: 반환 값을 추가했습니다. 새 Path 인스턴스를 반환합니다.
-
Path.
resolve
(strict=False)¶ 심볼릭 링크를 결정하여, 경로를 절대적으로 만듭니다. 새로운 경로 객체가 반환됩니다:
>>> p = Path() >>> p PosixPath('.') >>> p.resolve() PosixPath('/home/antoine/pathlib')
“
..
” 구성 요소도 제거됩니다 (이것이 이렇게 하는 유일한 메서드입니다):>>> p = Path('docs/../setup.py') >>> p.resolve() PosixPath('/home/antoine/pathlib/setup.py')
경로가 존재하지 않고 strict가
True
이면,FileNotFoundError
가 발생합니다. strict가False
이면, 경로는 가능한 만큼 결정되고 나머지는 존재하는지 확인하지 않고 추가됩니다. 경로를 결정하는 도중 무한 루프를 만나면,RuntimeError
가 발생합니다.버전 3.6에 추가: strict 인자 (3.6 이전 동작은 엄격(strict)합니다).
-
Path.
rglob
(pattern)¶ 이것은 주어진 상대 pattern 앞에 “
**/
”가 추가된Path.glob()
을 호출하는 것과 같습니다:>>> sorted(Path().rglob("*.py")) [PosixPath('build/lib/pathlib.py'), PosixPath('docs/conf.py'), PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')]
인자
self
,pattern
으로 감사 이벤트pathlib.Path.rglob
을 발생시킵니다.
-
Path.
rmdir
()¶ 이 디렉터리를 제거합니다. 디렉터리는 비어 있어야 합니다.
-
Path.
samefile
(other_path)¶ 이 경로가 other_path와 같은 파일을 가리키는지를 반환합니다. other_path는 Path 객체이거나 문자열일 수 있습니다. 의미는
os.path.samefile()
과os.path.samestat()
과 유사합니다.어떤 이유로 파일에 액세스할 수 없으면
OSError
가 발생할 수 있습니다.>>> p = Path('spam') >>> q = Path('eggs') >>> p.samefile(q) False >>> p.samefile('spam') True
버전 3.5에 추가.
-
Path.
symlink_to
(target, target_is_directory=False)¶ 이 경로를 target에 대한 심볼릭 링크로 만듭니다. 윈도우에서, 링크의 대상이 디렉터리이면 target_is_directory는 참(기본값
False
)이어야 합니다. POSIX에서, target_is_directory의 값이 무시됩니다.>>> p = Path('mylink') >>> p.symlink_to('setup.py') >>> p.resolve() PosixPath('/home/antoine/pathlib/setup.py') >>> p.stat().st_size 956 >>> p.lstat().st_size 8
참고
인자의 순서(링크, 대상)는
os.symlink()
와 반대입니다.
-
Path.
hardlink_to
(target)¶ Make this path a hard link to the same file as target.
참고
The order of arguments (link, target) is the reverse of
os.link()
’s.버전 3.10에 추가.
-
Path.
link_to
(target)¶ Make target a hard link to this path.
경고
This function does not make this path a hard link to target, despite the implication of the function and argument names. The argument order (target, link) is the reverse of
Path.symlink_to()
andPath.hardlink_to()
, but matches that ofos.link()
.버전 3.8에 추가.
버전 3.10부터 폐지: This method is deprecated in favor of
Path.hardlink_to()
, as the argument order ofPath.link_to()
does not match that ofPath.symlink_to()
.
-
Path.
touch
(mode=0o666, exist_ok=True)¶ 이 지정된 경로에 파일을 만듭니다. mode가 제공되면, 프로세스의
umask
값과 결합하여 파일 모드와 액세스 플래그를 결정합니다. 파일이 이미 존재하면, exist_ok가 참일 때 함수가 성공하고 (그리고 수정 시간이 현재 시각으로 갱신됩니다), 그렇지 않으면FileExistsError
가 발생합니다.
-
Path.
unlink
(missing_ok=False)¶ 이 파일이나 심볼릭 링크를 제거합니다. 경로가 디렉터리를 가리키면,
Path.rmdir()
을 대신 사용하십시오.missing_ok가 거짓(기본값)이면, 경로가 없을 때
FileNotFoundError
가 발생합니다.missing_ok가 참이면,
FileNotFoundError
예외는 무시됩니다 (POSIXrm -f
명령과 같은 동작).버전 3.8에서 변경: missing_ok 매개 변수가 추가되었습니다.
-
Path.
write_bytes
(data)¶ 가리키는 파일을 바이너리 모드로 열고, data를 쓴 다음, 파일을 닫습니다:
>>> p = Path('my_binary_file') >>> p.write_bytes(b'Binary file contents') 20 >>> p.read_bytes() b'Binary file contents'
같은 이름의 기존 파일을 덮어씁니다.
버전 3.5에 추가.
-
Path.
write_text
(data, encoding=None, errors=None, newline=None)¶ 가리키는 파일을 텍스트 모드로 열고, data를 쓴 다음, 파일을 닫습니다:
>>> p = Path('my_text_file') >>> p.write_text('Text file contents') 18 >>> p.read_text() 'Text file contents'
같은 이름의 기존 파일을 덮어씁니다. 선택적 매개 변수는
open()
에서와 같은 의미입니다.버전 3.5에 추가.
버전 3.10에서 변경: The newline parameter was added.
os
모듈에 있는 도구와 대조¶
아래는 다양한 os
함수를 해당 PurePath
/Path
대응 물에 매핑하는 표입니다.
참고
Not all pairs of functions/methods below are equivalent. Some of them,
despite having some overlapping use-cases, have different semantics. They
include os.path.abspath()
and Path.resolve()
,
os.path.relpath()
and PurePath.relative_to()
.
Footnotes
- 1
os.path.abspath()
does not resolve symbolic links whilePath.resolve()
does.- 2
PurePath.relative_to()
requiresself
to be the subpath of the argument, butos.path.relpath()
does not.